What is a Marker Interface in Java?
- Sahadev Bite
- Dec 30, 2024
- 3 min read
A marker interface is an interface that has no methods or fields. It is used to provide metadata or tagging information to a class. By implementing a marker interface, a class conveys to the compiler or runtime system that it should be treated in a specific way.
Characteristics of a Marker Interface
No Methods or Fields:
A marker interface does not define any behavior.
Example
public interface Serializable {
// No methods or fields
}
Metadata Provider:
Acts as a marker or tag for a class, indicating that the class possesses certain properties or behaviors.
Compiler or Runtime Role:
The compiler or runtime checks if a class implements the marker interface to determine specific behavior.
Examples of Marker Interfaces in Java
Serializable:
Marks a class as capable of being serialized.
Serialization converts an object into a byte stream for storage or transmission.
Classes that do not implement Serializable will throw a NotSerializableException during serialization.
Cloneable:
Marks a class as capable of being cloned using the clone() method.
If a class does not implement Cloneable, calling clone() on its object will throw a CloneNotSupportedException.
Remote:
Used in Java RMI (Remote Method Invocation).
Marks a class as eligible for remote method calls.
Why Use Marker Interfaces?
1. Metadata Tagging
Marker interfaces are a way to tag a class with metadata, indicating that it has specific capabilities or properties.
Example: By implementing Serializable, a class indicates that it can be serialized.
2. Type Checking
Marker interfaces enable compile-time type checking.
Example: The instanceof operator can be used to check if an object implements a marker interface.
3. Runtime Behavior
Marker interfaces allow the JVM or frameworks to apply specific behavior to classes at runtime.
Example: The JVM checks if a class implements Serializable before attempting serialization.
4. Backward Compatibility
Before annotations were introduced in Java 5, marker interfaces were the primary way to provide metadata for classes.
How Do Marker Interfaces Work?
Marker interfaces work through:
Reflection:
The JVM or libraries use reflection to check if a class implements a specific marker interface.
Instance Checking:
Using instanceof to determine if an object implements the marker interface.
Example: Marker Interface in Action
Without Marker Interface
If a class does not implement Serializable, serialization will fail:
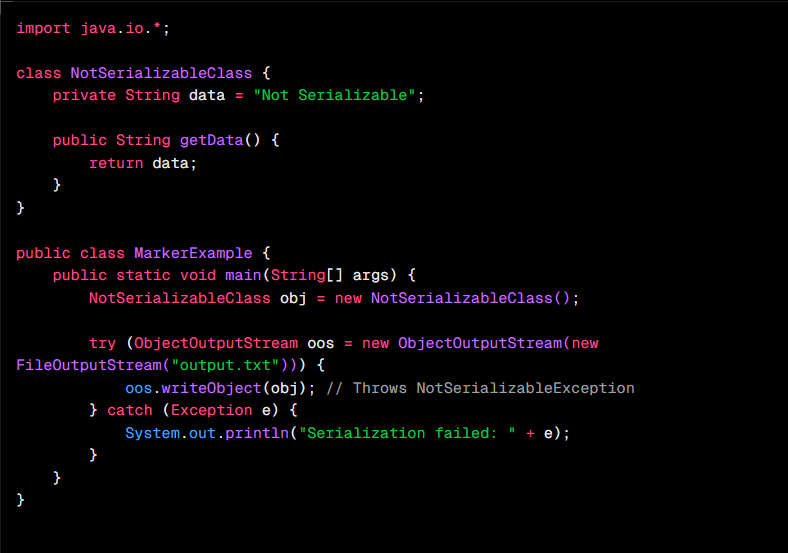
With Marker Interface
If the class implements Serializable, serialization works:
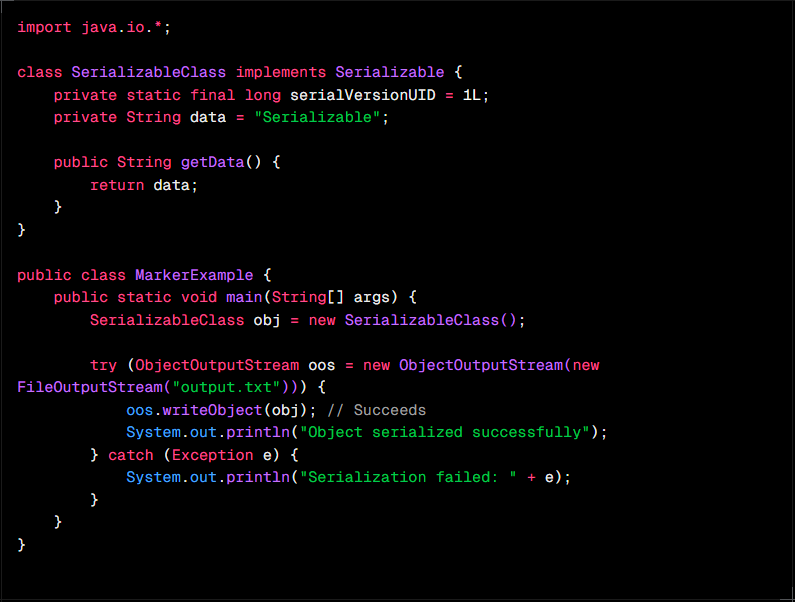
Advantages of Marker Interfaces
Simple and Easy to Use:
No additional code is required to implement a marker interface.
Type Safety:
Ensures compile-time type checking, reducing runtime errors.
Backward Compatibility:
Works seamlessly with older Java versions.
Minimal Overhead:
No additional runtime cost as no methods are implemented.
Limitations of Marker Interfaces
No Runtime Data:
Cannot provide additional information or metadata at runtime.
Annotations Are More Flexible:
Modern Java frameworks prefer annotations, as they can carry metadata and are more versatile.
Marker Interfaces vs Annotations
Aspect | Marker Interface | Annotation |
Introduced In | Java 1.0 | Java 5 |
Purpose | Tags a class for specific behavior | Tags a class, method, or field for metadata |
Methods or Fields | Cannot define methods or fields | Can include attributes (metadata) |
Example | Serializable, Cloneable | @Override, @Deprecated |
Runtime Processing | Requires reflection or type checking (instanceof) | Easier runtime processing using annotations |
Key Takeaways
A marker interface is an interface with no methods or fields, used to mark a class for specific behavior.
Marker interfaces are still useful for scenarios requiring type checking or backward compatibility.
Annotations have largely replaced marker interfaces in modern Java development due to their flexibility and metadata capabilities.
Comments